How to make your own online Code Compiler Platform in 30 minutes
For this Demo Tute we will be showcasing just Python:
Install Node.js from https://nodejs.org/en/ this will also install NPM on your Server\System.
NPM will be used to install various packages given below to make this work:
Install compileX using command given below on cmd:
Install express using command given below on cmd:
Install body-parser using the command given below on cmd:
Install Python on your Server\System as this will be used by our web app to execute code.
Set Python in Path variable so that you can execute it from anywhere from cmd without traversing directories again and again.
Add code given below to notepad and save it as TestPython.js in some directory.
In the same directory where you have saved TestPython.js file create a .html file with name as index.html and add the following code to it :
Next execute TestPython.js file using command on cmd and keep it spinning in the background:
Now goto your webbrowser and visit the url http://localhost:8080/ as shown in the screenshot below :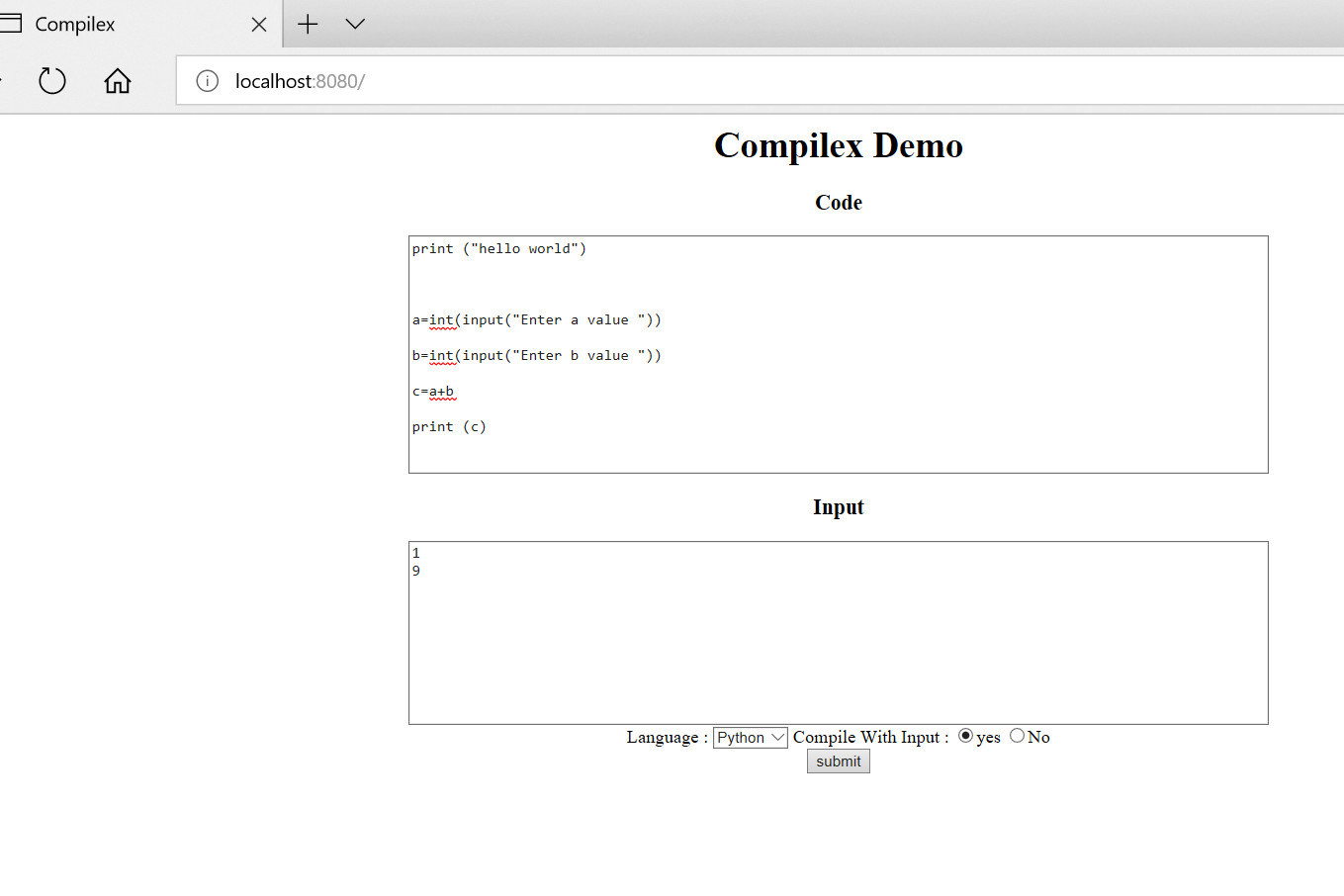
Each
execution creates a temp file in the temp folder created by compilex
for us which will be require cleanup over period of time.
Enter
the python code snippet given below in code input box and 2 parameters
separated by new line in input box select Python as Language select Yes
radio button in compile with input option and hit submit :
If everything works fine you will get a output in JSON format as shown below :
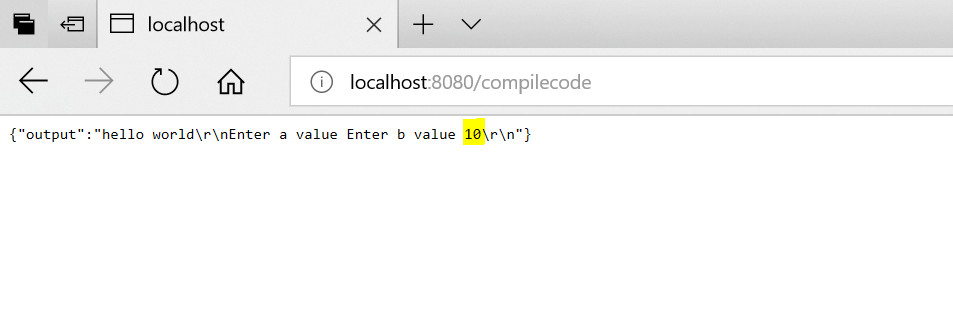
Further Reading\References:
https://www.npmjs.com/package/compilex
https://www.npmjs.com/package/express
Where and how it can be used ?I am sure you might have heard about sites like Hackerrank (Formerly: Interview street),Codechef etc which are used by industry giants like Facebook etc to hire candidates by throwing them a realtime coding challenge,
with this we will be able to enable a similar functionality in any website itself like the ones used by recruiters to hire people with right
skills easily when they are looking for coders.
With some further development and CSS makeup we can create a good looking platform
for people to code and compete for the Job and get ranked so that when
the opening for some JOB comes up recruiters and candidates don't have
search for each other as they will be available right there along with
data and there rank to prove there qualifications and validity which in
turn will also reduce time taken by a requisition to get fulfilled.
We can in the same way rank recruiters based on the incentives and other
extra facilities they provide etc and the number of people they actually
hire over number of vacancies they publish on platform so that quality
recruiters meet quality candidates,which will motivate both parties to
keep there standards high !
npm i compilex
npm i express
npm i body-parser
var express = require('express'); var path = require('path'); var app = express(); var bodyParser = require('body-parser'); app.use(bodyParser.urlencoded({ extended: true })); app.use(bodyParser.json()); //compileX var compiler = require('compilex'); var option = {stats : true}; compiler.init(option); app.get('/' , function (req , res ) { res.sendFile( __dirname + "/index.html"); }); app.post('/compilecode' , function (req , res ) { var code = req.body.code; var input = req.body.input; var inputRadio = req.body.inputRadio; var lang = req.body.lang; if((lang === "C") || (lang === "C++")) { if(inputRadio === "true") { var envData = { OS : "windows" , cmd : "g++"}; compiler.compileCPPWithInput(envData , code ,input , function (data) { if(data.error) { res.send(data.error); } else { res.send(data.output); } }); } else { var envData = { OS : "windows" , cmd : "g++"}; compiler.compileCPP(envData , code , function (data) { if(data.error) { res.send(data.error); } else { res.send(data.output); } }); } } if(lang === "Java") { if(inputRadio === "true") { var envData = { OS : "windows" }; console.log(code); compiler.compileJavaWithInput( envData , code , function(data){ res.send(data); }); } else { var envData = { OS : "windows" }; console.log(code); compiler.compileJavaWithInput( envData , code , input , function(data){ res.send(data); }); } } if( lang === "Python") { if(inputRadio === "true") { var envData = { OS : "windows"}; compiler.compilePythonWithInput(envData , code , input , function(data){ res.send(data); }); } else { var envData = { OS : "windows"}; compiler.compilePython(envData , code , function(data){ res.send(data); }); } } if( lang === "CS") { if(inputRadio === "true") { var envData = { OS : "windows"}; compiler.compileCSWithInput(envData , code , input , function(data){ res.send(data); }); } else { var envData = { OS : "windows"}; compiler.compileCS(envData , code , function(data){ res.send(data); }); } } if( lang === "VB") { if(inputRadio === "true") { var envData = { OS : "windows"}; compiler.compileVBWithInput(envData , code , input , function(data){ res.send(data); }); } else { var envData = { OS : "windows"}; compiler.compileVB(envData , code , function(data){ res.send(data); }); } } }); app.get('/fullStat' , function(req , res ){ compiler.fullStat(function(data){ res.send(data); }); }); app.listen(8080);
<html> <head> <title>Compilex</title> </head> <body> <center> <h1>Compilex Demo</h1> <form id="myform" name="myform" method="post" action="compilecode"> <h3>Code</h3> <textarea rows="13" cols="100" id="code" name="code" ></textarea> <br/> <h3>Input</h3> <textarea rows="10" cols="100" id="input" name="input" ></textarea> <br/> Language : <select name="lang"> <option value="C">C</option> <option value="C++">C++</option> <option value="Java">Java</option> <option value="Python">Python</option> <option value="CS">C#</option> <option value="VB">VB</option> </select> Compile With Input : <input type="radio" name="inputRadio" id="inputRadio" value="true"/>yes <input type="radio" name="inputRadio" id="inputRadio" value="false"/>No <br/> <input type="submit" value="submit" name="submit"/> </form> </center> </body> </html>
node TestPython.js
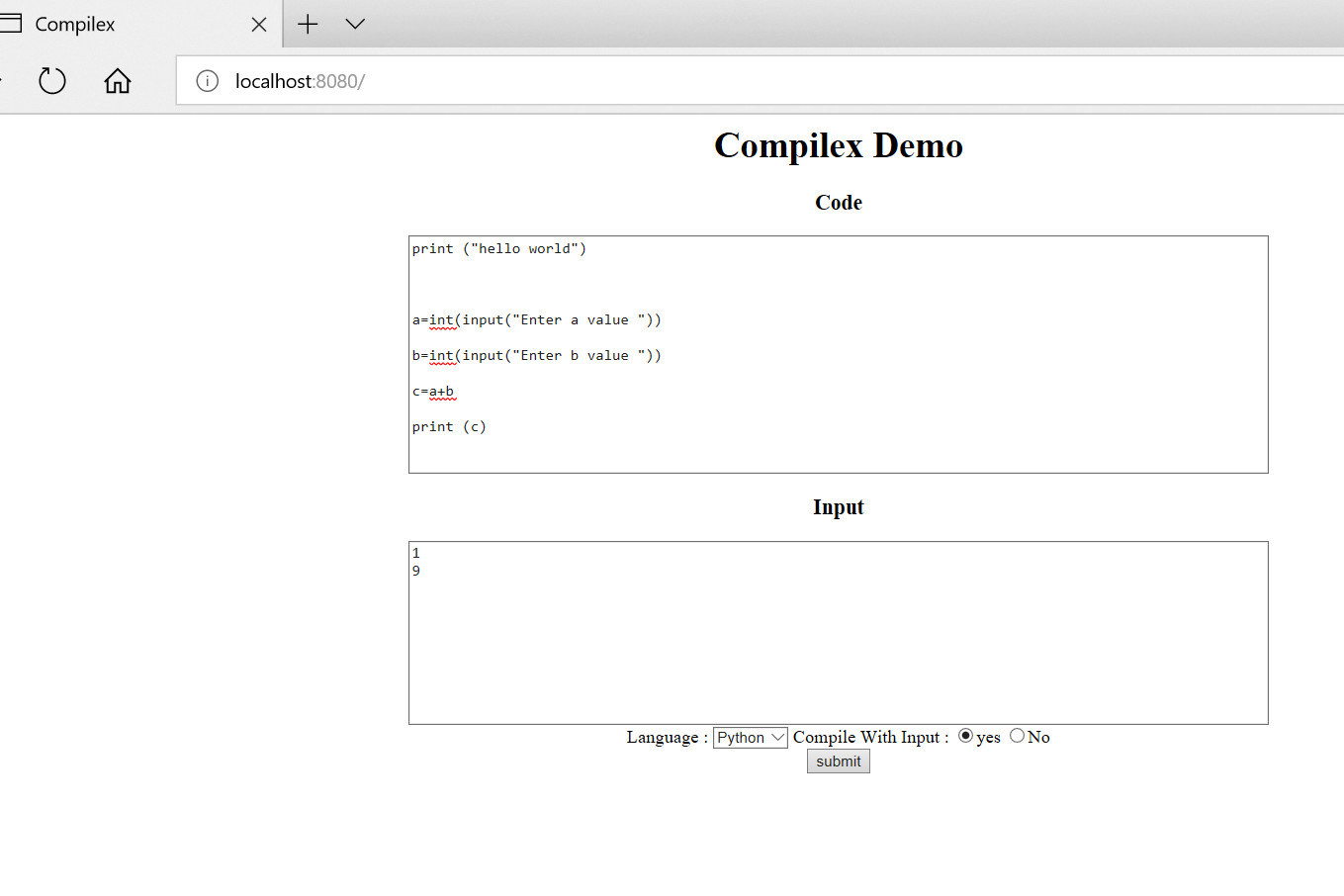
print ("hello world") a=int(input("Enter a value ")) b=int(input("Enter b value ")) c=a+b print (c)
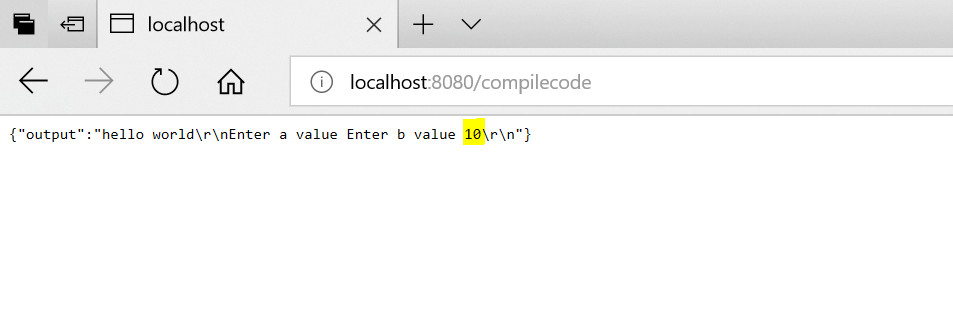
Further Reading\References:
https://www.npmjs.com/package/compilex
https://www.npmjs.com/package/express
Where and how it can be used ?
Comments
Post a Comment
Note:Please be gentle while commenting and avoid spamming as comment are anyways moderated thank you.